Aug
Highcharts Implementation in Angular
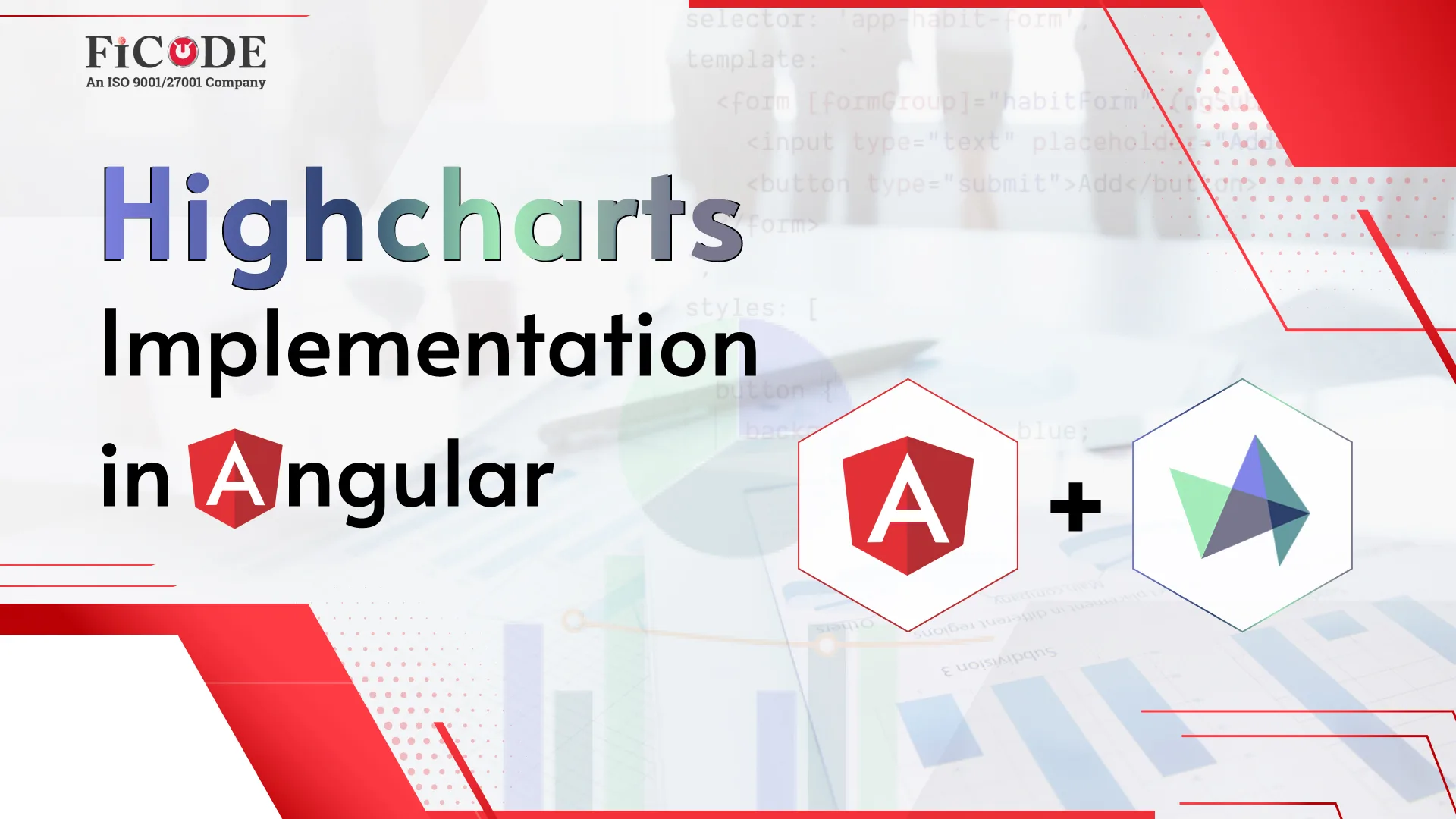
If you want to create stunning charts and graphs in your Angular applications to make data look visually appealing, you’re in the right place. We’ll guide you through the process of integrating Highcharts, a powerful JavaScript charting library, with the popular Angular framework.
Implementing the Highcharts in an Angular application involves several steps, including installing Highcharts package, creating the chart configuration, creating a component, and integrating the chart within the component.
These are the below-mentioned that states why we use the Highcharts:
1) Highcharts is used to visualize the Tabular data in the form of high-quality Charts and Graphs that are more suitable for the graphical user interface; by using this, the user can easily read and understand the data.
2) We can also visualize the live data points seamlessly without refreshing the page/chart.
3) We can also add several types of filters in the Highcharts to filter the graphical data.
1) Install Highcharts package:
First, you need to install the Highcharts library in your Angular project. You can use npm or yarn for this purpose. Open your terminal and run:
npm install highcharts
2) Create a Component:
Create a new Angular Component in which you’ll be implementing the Highcharts chart. You can generate a new component using Angular CLI:
ng generate component ComponentName
3) Import Highcharts and implementation:
Edit the newly added TS file component-name.component.ts file and import Highcharts at the top of the file:
import * as Highcharts from 'highcharts';
3.1) Chart Series:
A series refers to the data points and their visual representation on a chart. It also represents a set of data that you want to display using a specific type of chart, such as a line chart, bar chart, pie chart.
series: [{ type: 'line', name: 'Temperature', data: [ [0, 22] color: '#0077FF' }]
3.2) Add live streaming data:
For adding live streaming in any series of charts we will use “addPoint” function from the library itself. “addPoint” function allows you to add a new data point to an existing series
chart.series[0].addPoint([xValue, yValue]);
3.3) Replace the chart Series Data:
To replace the entire data array of a series with a new set of data points we use the “setData” function.
var newData = [ [xValue1, yValue1], [xValue2, yValue2], ]; chart.series[0].setData(newData);
3.4) GapSize in Chart:
The gap size property is used to control the spacing between data points in a series. It allows you to specify the gap size as a percentage of the point’s width. It is specifically useful when you have irregularly spaced data points, and you want to control the spacing between them
series: [{ type: 'line', name: 'Gap Size Example', data: [ [0, 5], [1, 8], [3, 12], // Notice the gap between x-values 1 and 3 [4, 10], [5, 15] ], gapSize: 0.2, // Set the gap size as 20% of the point's width }]
4) Set up Chart Data:
In your component’s TypeScript file (component-name.component.ts), you can define the data you want to visualize in your chart.
renderChart(): void { Highcharts.chart('chart-container', { title: { text: 'Sample Chart' }, series: [{ type: 'line', name: 'Data Series', data: [1, 2, 3, 4, 5] }] }); }
5) HTML Template:
In your component’s HTML file (component-name.component.html), add an element to serve as the container for your chart:
<div id="chart-container"></div>
6) Add Component to a View:
Finally, you can include your chart component in your application by adding the following line to the desired template, such as app.component.html:
<app-chart></app-chart> import { Component, OnInit, AfterViewInit } from '@angular/core'; import * as Highcharts from 'highcharts'; @Component({ selector: 'app-sales-chart', templateUrl: './sales-chart.component.html', styleUrls: ['./sales-chart.component.css'] }) export class SalesChartComponent implements OnInit, AfterViewInit { // ... (dummy dataset here) constructor() { } ngOnInit(): void { } ngAfterViewInit(): void { this.createChart(); } createChart(): void { Highcharts.chart('chartContainer', { chart: { type: 'column' }, title: { text: 'Monthly Sales' }, xAxis: { categories: this.data.map(item => item.month) }, yAxis: { title: { text: 'Sales' } }, series: [{ name: 'Sales', data: this.data.map(item => item.sales) }] }); } }